In this example, the following modules: Script, TypeData, WorkPiece, PowerSupply, Multimeter and HighVoltageTester are all on the same level within the module tree.
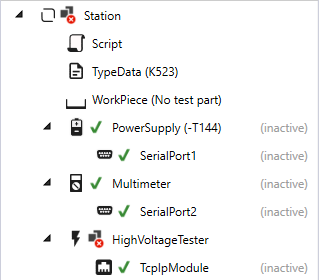
These modules can therefore be accessed directly from the start file:
PowerSupply.SetVoltage(14.0)